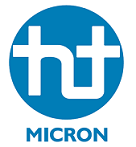 |
HT32SX Generic Push Button
Generic Push Button application for HT32SX
|
Go to the documentation of this file.
31 #ifndef SIGFOX_MONARCH_API_H
32 #define SIGFOX_MONARCH_API_H
86 #define MAX_MONARCH_PATTERN_PER_FREQUENCY_SEARCH 2
Definition: sigfox_monarch_api.h:57
Definition: sigfox_monarch_api.h:90
Definition: sigfox_monarch_api.h:62
Definition: sigfox_monarch_api.h:65
Definition: sigfox_monarch_api.h:56
Definition: sigfox_monarch_api.h:82
sfx_bitfield_rc_enum_t
Definition: sigfox_monarch_api.h:51
Definition: sigfox_monarch_api.h:44
Definition: sigfox_monarch_api.h:110
sfx_pattern_enum_t
Definition: sigfox_monarch_api.h:72
Definition: sigfox_monarch_api.h:46
Definition: sigfox_monarch_api.h:61
Definition: sigfox_monarch_api.h:58
sfx_error_t SIGFOX_MONARCH_API_stop_rc_scan(void)
This function stops a RC scan which is on going.
Definition: sigfox_monarch_api.h:59
sfx_monarch_listening_mode_t
Definition: sigfox_monarch_api.h:100
Definition: sigfox_monarch_api.h:81
Definition: sigfox_monarch_api.h:105
sfx_timer_unit_enum_t
Definition: sigfox_monarch_api.h:39
Definition: sigfox_monarch_api.h:79
Definition: sigfox_monarch_api.h:60
sfx_error_t SIGFOX_MONARCH_API_execute_rc_scan(sfx_u8 rc_capabilities_bit_mask, sfx_u16 timer, sfx_timer_unit_enum_t unit, sfx_u8(*app_callback_handler)(sfx_u8 rc_bit_mask, sfx_s16 rssi))
This function executes a scan of the air to detect a Sigfox Beacon. It will return the RC enum value ...
Definition: sigfox_monarch_api.h:80
Definition: sigfox_monarch_api.h:85
Definition: sigfox_monarch_api.h:45
Definition: sigfox_monarch_api.h:43