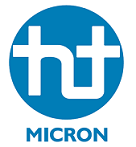 |
HT32SX Generic Push Button
Generic Push Button application for HT32SX
|
Go to the documentation of this file.
91 #define SFX_ERR_NONE (sfx_u8)(0x00)
99 #define SFX_ERR_API_OPEN (sfx_u8)(0x10)
100 #define SFX_ERR_API_OPEN_STATE (sfx_u8)(0x11)
101 #define SFX_ERR_API_OPEN_GET_NVMEM_MEMORY_OVERLAP (sfx_u8)(0x12)
102 #define SFX_ERR_API_OPEN_RC_PTR (sfx_u8)(0x13)
103 #define SFX_ERR_API_OPEN_MACRO_CHANNEL_WIDTH (sfx_u8)(0x14)
105 #define SFX_ERR_API_CLOSE_FREE (sfx_u8)(0x20)
106 #define SFX_ERR_API_CLOSE_STATE (sfx_u8)(0x21)
108 #define SFX_ERR_API_SEND_FRAME_DATA_LENGTH (sfx_u8)(0x30)
109 #define SFX_ERR_API_SEND_FRAME_RESPONSE_PTR (sfx_u8)(0x31)
110 #define SFX_ERR_API_SEND_FRAME_DELAY_OOB_ACK (sfx_u8)(0x32)
111 #define SFX_ERR_API_SEND_FRAME_DATA_PTR (sfx_u8)(0x33)
113 #define SFX_ERR_API_SEND_BIT_RESPONSE_PTR (sfx_u8)(0x34)
114 #define SFX_ERR_API_SEND_OOB_TYPE (sfx_u8)(0x35)
117 #define SFX_ERR_API_SET_STD_CONFIG_CARRIER_SENSE_CONFIG (sfx_u8)(0x40)
118 #define SFX_ERR_API_SET_STD_CONFIG_FH_CHANNELS (sfx_u8)(0x41)
120 #define SFX_ERR_API_SEND_TEST_FRAME_DEVICE_ID (sfx_u8)(0x50)
121 #define SFX_ERR_API_SEND_TEST_FRAME_STATE (sfx_u8)(0x51)
122 #define SFX_ERR_API_SEND_TEST_FRAME_DATA_LENGTH (sfx_u8)(0x52)
123 #define SFX_ERR_API_SEND_TEST_FRAME_DATA_PTR (sfx_u8)(0x53)
124 #define SFX_ERR_API_SEND_TEST_STORE_NVM (sfx_u8)(0x54)
126 #define SFX_ERR_API_RECEIVE_TEST_FRAME_DEVICE_ID (sfx_u8)(0x55)
127 #define SFX_ERR_API_RECEIVE_TEST_FRAME_STATE (sfx_u8)(0x56)
129 #define SFX_ERR_API_START_CONTINUOUS_TRANSMISSION (sfx_u8)(0x57)
130 #define SFX_ERR_API_START_CONTINUOUS_TRANSMISSION_STATE (sfx_u8)(0x58)
131 #define SFX_ERR_API_STOP_CONTINUOUS_TRANSMISSION (sfx_u8)(0x59)
132 #define SFX_ERR_API_STOP_CONTINUOUS_TRANSMISSION_STATE (sfx_u8)(0x5A)
134 #define SFX_ERR_API_GET_INITIAL_PAC (sfx_u8)(0x5B)
135 #define SFX_ERR_API_GET_VERSION (sfx_u8)(0x5C)
136 #define SFX_ERR_API_GET_VERSION_WRONG_TYPE (sfx_u8)(0x5D)
137 #define SFX_ERR_API_SWITCH_PUBLIC_KEY (sfx_u8)(0x5E)
139 #define SFX_ERR_INT_EXECUTE_COM_SEQUENCE_STATE (sfx_u8)(0x60)
140 #define SFX_ERR_INT_EXECUTE_COM_SEQUENCE_NVM_STORAGE_MESSAGE (sfx_u8)(0x61)
141 #define SFX_ERR_INT_EXECUTE_COM_SEQUENCE_NVM_STORAGE_ACK (sfx_u8)(0x62)
142 #define SFX_ERR_INT_EXECUTE_COM_SEQUENCE_NVM_STORAGE_RCSYNC (sfx_u8)(0x63)
143 #define SFX_ERR_INT_EXECUTE_COM_SEQUENCE_DELAY_OOB_ACK (sfx_u8)(0x64)
145 #define SFX_ERR_INT_PROCESS_UPLINK_START_TIMER_FH_IN_DL (sfx_u8)(0x70)
146 #define SFX_ERR_INT_PROCESS_UPLINK_WAIT_FOR_END_TIMER_FH_IN_DL (sfx_u8)(0x71)
147 #define SFX_ERR_INT_PROCESS_UPLINK_TIMER_FH (sfx_u8)(0x72)
148 #define SFX_ERR_INT_PROCESS_UPLINK_WAIT_FOR_END_TIMER_FH (sfx_u8)(0x73)
149 #define SFX_ERR_INT_PROCESS_UPLINK_DELAY_INTERFRAME (sfx_u8)(0x74)
150 #define SFX_ERR_INT_PROCESS_UPLINK_TIMER_DOWNLINK (sfx_u8)(0x75)
151 #define SFX_ERR_INT_PROCESS_UPLINK_CS_RETRY (sfx_u8)(0x76)
152 #define SFX_ERR_INT_PROCESS_UPLINK_CS_RETRY_START_TIMER (sfx_u8)(0x77)
153 #define SFX_ERR_INT_PROCESS_UPLINK_CS_RETRY_STOP_TIMER (sfx_u8)(0x78)
154 #define SFX_ERR_INT_PROCESS_UPLINK_CS_RETRY_DELAY_ATTEMPT (sfx_u8)(0x79)
155 #define SFX_ERR_INT_PROCESS_UPLINK_CS_REPETITION (sfx_u8)(0x7A)
156 #define SFX_ERR_INT_PROCESS_UPLINK_CS_REPETITION_START_TIMER (sfx_u8)(0x7B)
157 #define SFX_ERR_INT_PROCESS_UPLINK_CS_REPETITION_STOP_TIMER (sfx_u8)(0x7C)
158 #define SFX_ERR_INT_PROCESS_UPLINK_CS_REPETITION_STOP_TIMER_2 (sfx_u8)(0x7D)
159 #define SFX_ERR_INT_PROCESS_UPLINK_CS_TIMEOUT (sfx_u8)(0x7E)
161 #define SFX_ERR_INT_BUILD_FRAME_SE (sfx_u8)(0x90)
162 #define SFX_ERR_INT_BUILD_FRAME (sfx_u8)(0x91)
163 #define SFX_ERR_INT_BUILD_FRAME_OOB_SERVICE (sfx_u8)(0x92)
164 #define SFX_ERR_INT_BUILD_FRAME_OOB_DOWNLINK_ACK (sfx_u8)(0x93)
165 #define SFX_ERR_INT_BUILD_FRAME_OOB_REPEATER_STATUS (sfx_u8)(0x94)
166 #define SFX_ERR_INT_BUILD_FRAME_OOB_RC_SYNC (sfx_u8)(0x95)
167 #define SFX_ERR_INT_BUILD_FRAME_PAYLOAD_CRYPTED (sfx_u8)(0x96)
169 #define SFX_ERR_INT_SEND_SINGLE_FRAME (sfx_u8)(0x97)
170 #define SFX_ERR_INT_PROCESS_DOWNLINK (sfx_u8)(0x98)
172 #define SFX_ERR_INT_GET_DEVICE_ID (sfx_u8)(0x99)
173 #define SFX_ERR_INT_GET_RECEIVED_FRAMES (sfx_u8)(0x9A)
174 #define SFX_ERR_INT_GET_RECEIVED_FRAMES_TIMEOUT (sfx_u8)(0x9B)
175 #define SFX_ERR_INT_GET_RECEIVED_FRAMES_WAIT_NOT_EXECUTED (sfx_u8)(0x9C)
177 #define SFX_ERR_INT_GET_DEVICE_INFO (sfx_u8)(0x9D)
178 #define SFX_ERR_INT_GET_DEVICE_INFO_CRC (sfx_u8)(0x9E)
179 #define SFX_ERR_INT_GET_DEVICE_INFO_CERTIFICATE (sfx_u8)(0x9F)
182 #define SFX_ERR_API_SET_RC_SYNC_PERIOD (sfx_u8)(0xB0)
183 #define SFX_ERR_API_SET_RC_SYNC_PERIOD_VALUE (sfx_u8)(0xB1)
185 #define SFX_ERR_MONARCH_API_EXECUTE_RC_SCAN_STATE (sfx_u8)(0xB2)
186 #define SFX_ERR_MONARCH_API_EXECUTE_RC_SCAN (sfx_u8)(0xB3)
187 #define SFX_ERR_MONARCH_API_EXECUTE_RC_SCAN_NULL_CALLBACK (sfx_u8)(0xB4)
188 #define SFX_ERR_MONARCH_API_STOP_RC_SCAN_STATE (sfx_u8)(0xB5)
189 #define SFX_ERR_MONARCH_API_STOP_RC_SCAN (sfx_u8)(0xB6)
190 #define SFX_ERR_CALLBACK_MONARCH_SCAN_TIMEOUT_CB_STATE (sfx_u8)(0xB7)
191 #define SFX_ERR_CALLBACK_MONARCH_SCAN_TIMEOUT (sfx_u8)(0xB8)
192 #define SFX_ERR_CALLBACK_MONARCH_PATTERN_FREQUENCY_RESULT_STATE (sfx_u8)(0xB9)
193 #define SFX_ERR_CALLBACK_MONARCH_PATTERN_FREQUENCY_RESULT (sfx_u8)(0xBA)
194 #define SFX_ERR_CALLBACK_MONARCH_PATTERN_FREQUENCY_RESULT_WRONG_PATTERN (sfx_u8)(0xBB)
195 #define SFX_ERR_CALLBACK_MONARCH_PATTERN_FREQUENCY_RESULT_WRONG_FREQ (sfx_u8)(0xBC)
204 #define SFX_ERR_INT_DOWNLINK_CONFIGURATION (sfx_u8)(0xE0)
219 #define RC1_OPEN_UPLINK_CENTER_FREQUENCY (sfx_u32)(868130000)
220 #define RC1_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(869525000)
221 #define RC1_MACRO_CHANNEL_WIDTH (sfx_u32)(192000)
222 #define RC1_UPLINK_MODULATION SFX_DBPSK_100BPS
223 #define RC1_UPLINK_SPECTRUM_ACCESS SFX_DC
225 #define RC2_OPEN_UPLINK_START_OF_TABLE (sfx_u32)(902200000)
226 #define RC2_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(905200000)
227 #define RC2_MACRO_CHANNEL_WIDTH (sfx_u32)(192000)
228 #define RC2_UPLINK_MODULATION SFX_DBPSK_600BPS
229 #define RC2_UPLINK_SPECTRUM_ACCESS SFX_FH
230 #define RC2_SET_STD_CONFIG_LM_WORD_0 (sfx_u32)0x000001FF
231 #define RC2_SET_STD_CONFIG_LM_WORD_1 (sfx_u32)0x00000000
232 #define RC2_SET_STD_CONFIG_LM_WORD_2 (sfx_u32)0x00000000
233 #define RC2_SET_STD_TIMER_ENABLE (sfx_bool)(SFX_TRUE)
234 #define RC2_SET_STD_TIMER_DISABLE (sfx_bool)(SFX_FALSE)
235 #define RC2_SET_STD_CONFIG_SM_WORD_0 (sfx_u32)0x00000001
236 #define RC2_SET_STD_CONFIG_SM_WORD_1 (sfx_u32)0x00000000
237 #define RC2_SET_STD_CONFIG_SM_WORD_2 (sfx_u32)0x00000000
239 #define RC3A_OPEN_CS_CENTER_FREQUENCY (sfx_u32)(923200000)
240 #define RC3A_OPEN_CS_BANDWIDTH (sfx_u32)(200000)
241 #define RC3A_OPEN_UPLINK_CENTER_FREQUENCY (sfx_u32)(923200000)
242 #define RC3A_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(922200000)
243 #define RC3A_MACRO_CHANNEL_WIDTH (sfx_u32)(36000)
244 #define RC3A_UPLINK_MODULATION SFX_DBPSK_100BPS
245 #define RC3A_UPLINK_SPECTRUM_ACCESS SFX_LBT
246 #define RC3A_CS_THRESHOLD (sfx_s8)(-80)
248 #define RC3C_OPEN_CS_CENTER_FREQUENCY (sfx_u32)(923200000)
249 #define RC3C_OPEN_CS_BANDWIDTH (sfx_u32)(200000)
250 #define RC3C_OPEN_UPLINK_CENTER_FREQUENCY (sfx_u32)(923200000)
251 #define RC3C_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(922200000)
252 #define RC3C_MACRO_CHANNEL_WIDTH (sfx_u32)(192000)
253 #define RC3C_UPLINK_MODULATION SFX_DBPSK_100BPS
254 #define RC3C_UPLINK_SPECTRUM_ACCESS SFX_LBT
255 #define RC3C_CS_THRESHOLD (sfx_s8)(-80)
257 #define RC4_OPEN_UPLINK_START_OF_TABLE (sfx_u32)(902200000)
258 #define RC4_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(922300000)
259 #define RC4_MACRO_CHANNEL_WIDTH (sfx_u32)(192000)
260 #define RC4_UPLINK_MODULATION SFX_DBPSK_600BPS
261 #define RC4_UPLINK_SPECTRUM_ACCESS SFX_FH
262 #define RC4_SET_STD_CONFIG_LM_WORD_0 (sfx_u32)0x00000000
263 #define RC4_SET_STD_CONFIG_LM_WORD_1 (sfx_u32)0xF0000000
264 #define RC4_SET_STD_CONFIG_LM_WORD_2 (sfx_u32)0x0000001F
265 #define RC4_SET_STD_TIMER_ENABLE (sfx_bool)(SFX_TRUE)
266 #define RC4_SET_STD_TIMER_DISABLE (sfx_bool)(SFX_FALSE)
267 #define RC4_SET_STD_CONFIG_SM_WORD_0 (sfx_u32)0x00000000
268 #define RC4_SET_STD_CONFIG_SM_WORD_1 (sfx_u32)0x40000000
269 #define RC4_SET_STD_CONFIG_SM_WORD_2 (sfx_u32)0x00000000
271 #define RC5_OPEN_CS_CENTER_FREQUENCY (sfx_u32)(923300000)
272 #define RC5_OPEN_CS_BANDWIDTH (sfx_u32)(200000)
273 #define RC5_OPEN_UPLINK_CENTER_FREQUENCY (sfx_u32)(923300000)
274 #define RC5_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(922300000)
275 #define RC5_MACRO_CHANNEL_WIDTH (sfx_u32)(192000)
276 #define RC5_UPLINK_MODULATION SFX_DBPSK_100BPS
277 #define RC5_UPLINK_SPECTRUM_ACCESS SFX_LBT
278 #define RC5_CS_THRESHOLD (sfx_s8)(-65)
280 #define RC6_OPEN_UPLINK_CENTER_FREQUENCY (sfx_u32)(865200000)
281 #define RC6_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(866300000)
282 #define RC6_MACRO_CHANNEL_WIDTH (sfx_u32)(192000)
283 #define RC6_UPLINK_MODULATION SFX_DBPSK_100BPS
284 #define RC6_UPLINK_SPECTRUM_ACCESS SFX_DC
286 #define RC7_OPEN_UPLINK_CENTER_FREQUENCY (sfx_u32)(868800000)
287 #define RC7_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(869100000)
288 #define RC7_MACRO_CHANNEL_WIDTH (sfx_u32)(192000)
289 #define RC7_UPLINK_MODULATION SFX_DBPSK_100BPS
290 #define RC7_UPLINK_SPECTRUM_ACCESS SFX_DC
292 #define RC101_OPEN_UPLINK_CENTER_FREQUENCY (sfx_u32)(68862500)
293 #define RC101_OPEN_DOWNLINK_CENTER_FREQUENCY (sfx_u32)(72912500)
294 #define RC101_MACRO_CHANNEL_WIDTH (sfx_u32)(12500)
295 #define RC101_UPLINK_MODULATION SFX_DBPSK_100BPS
296 #define RC101_UPLINK_SPECTRUM_ACCESS SFX_DC
306 #define RC1 {RC1_OPEN_UPLINK_CENTER_FREQUENCY, RC1_OPEN_DOWNLINK_CENTER_FREQUENCY, RC1_MACRO_CHANNEL_WIDTH, RC1_UPLINK_MODULATION, RC1_UPLINK_SPECTRUM_ACCESS, {NA,NA,NA}}
307 #define RC2 {RC2_OPEN_UPLINK_START_OF_TABLE, RC2_OPEN_DOWNLINK_CENTER_FREQUENCY, RC2_MACRO_CHANNEL_WIDTH, RC2_UPLINK_MODULATION, RC2_UPLINK_SPECTRUM_ACCESS, {NA,NA,NA}}
308 #define RC3A {RC3A_OPEN_UPLINK_CENTER_FREQUENCY, RC3A_OPEN_DOWNLINK_CENTER_FREQUENCY, RC3A_MACRO_CHANNEL_WIDTH, RC3A_UPLINK_MODULATION, RC3A_UPLINK_SPECTRUM_ACCESS, {RC3A_OPEN_CS_CENTER_FREQUENCY,RC3A_OPEN_CS_BANDWIDTH, RC3A_CS_THRESHOLD}}
309 #define RC3C {RC3C_OPEN_UPLINK_CENTER_FREQUENCY, RC3C_OPEN_DOWNLINK_CENTER_FREQUENCY, RC3C_MACRO_CHANNEL_WIDTH, RC3C_UPLINK_MODULATION, RC3C_UPLINK_SPECTRUM_ACCESS, {RC3C_OPEN_CS_CENTER_FREQUENCY,RC3C_OPEN_CS_BANDWIDTH, RC3C_CS_THRESHOLD}}
311 #define RC4 {RC4_OPEN_UPLINK_START_OF_TABLE, RC4_OPEN_DOWNLINK_CENTER_FREQUENCY, RC4_MACRO_CHANNEL_WIDTH, RC4_UPLINK_MODULATION, RC4_UPLINK_SPECTRUM_ACCESS, {NA,NA,NA}}
312 #define RC5 {RC5_OPEN_UPLINK_CENTER_FREQUENCY, RC5_OPEN_DOWNLINK_CENTER_FREQUENCY, RC5_MACRO_CHANNEL_WIDTH, RC5_UPLINK_MODULATION, RC5_UPLINK_SPECTRUM_ACCESS, {RC5_OPEN_CS_CENTER_FREQUENCY,RC5_OPEN_CS_BANDWIDTH, RC5_CS_THRESHOLD}}
313 #define RC6 {RC6_OPEN_UPLINK_CENTER_FREQUENCY, RC6_OPEN_DOWNLINK_CENTER_FREQUENCY, RC6_MACRO_CHANNEL_WIDTH, RC6_UPLINK_MODULATION, RC6_UPLINK_SPECTRUM_ACCESS, {NA,NA,NA}}
314 #define RC7 {RC7_OPEN_UPLINK_CENTER_FREQUENCY, RC7_OPEN_DOWNLINK_CENTER_FREQUENCY, RC7_MACRO_CHANNEL_WIDTH, RC7_UPLINK_MODULATION, RC7_UPLINK_SPECTRUM_ACCESS, {NA,NA,NA}}
315 #define RC101 {RC101_OPEN_UPLINK_CENTER_FREQUENCY, RC101_OPEN_DOWNLINK_CENTER_FREQUENCY, RC101_MACRO_CHANNEL_WIDTH, RC101_UPLINK_MODULATION, RC101_UPLINK_SPECTRUM_ACCESS, {NA,NA,NA}}
317 #define RC2_LM_CONFIG {RC2_SET_STD_CONFIG_LM_WORD_0, RC2_SET_STD_CONFIG_LM_WORD_1, RC2_SET_STD_CONFIG_LM_WORD_2}
318 #define RC4_LM_CONFIG {RC4_SET_STD_CONFIG_LM_WORD_0, RC4_SET_STD_CONFIG_LM_WORD_1, RC4_SET_STD_CONFIG_LM_WORD_2}
320 #define RC2_SM_CONFIG {RC2_SET_STD_CONFIG_SM_WORD_0, RC2_SET_STD_CONFIG_SM_WORD_1, RC2_SET_STD_CONFIG_SM_WORD_2}
321 #define RC4_SM_CONFIG {RC4_SET_STD_CONFIG_SM_WORD_0, RC4_SET_STD_CONFIG_SM_WORD_1, RC4_SET_STD_CONFIG_SM_WORD_2}
323 #define RC3A_CONFIG {0x00000003,0x00001388,0x00000000}
324 #define RC3C_CONFIG {0x00000003,0x00001388,0x00000000}
325 #define RC5_CONFIG {0x00000003,0x00001388,0x00000000}
328 #define ID_LENGTH (sfx_u8)(4)
329 #define PAC_LENGTH (sfx_u8)(8)
367 }sfx_rx_state_enum_t;
378 SFX_MAX_OOB_LIST_SIZE,
424 SFX_NVMEM_BLOCK_SIZE = 7,
573 sfx_u8 customer_data_length,
574 sfx_u8* customer_response,
576 sfx_bool initiate_downlink_flag);
610 sfx_u8* customer_response,
612 sfx_bool initiate_downlink_flag);
714 sfx_bool timer_enable);
773 sfx_error_t
SIGFOX_API_send_test_frame(sfx_u32 frequency, sfx_u8* customer_data, sfx_u8 customer_data_length, sfx_bool initiate_downlink_flag);
Definition: sigfox_api.h:418
sfx_u32 open_cs_bandwidth
Definition: sigfox_api.h:466
Definition: sigfox_api.h:403
sfx_state_t
Definition: sigfox_api.h:385
sfx_error_t SIGFOX_API_close(void)
This function closes the library (Free the allocated memory of SIGFOX_API_open and close RF)
sfx_error_t SIGFOX_API_set_rc_sync_period(sfx_u16 rc_sync_period)
Set the period for transmission of RC Sync frame By default, when payload is encrypted,...
Definition: sigfox_api.h:343
sfx_error_t SIGFOX_API_send_bit(sfx_bool bit_value, sfx_u8 *customer_response, sfx_u8 tx_mode, sfx_bool initiate_downlink_flag)
Send a standard SIGFOX frame with null customer payload. This frame is the shortest that SIGFOX libra...
Definition: sigfox_api.h:351
Definition: sigfox_api.h:396
sfx_error_t SIGFOX_API_send_test_frame(sfx_u32 frequency, sfx_u8 *customer_data, sfx_u8 customer_data_length, sfx_bool initiate_downlink_flag)
This function builds a Sigfox Frame with the customer payload and send it at a specific frequency Use...
sfx_error_t SIGFOX_API_start_continuous_transmission(sfx_u32 frequency, sfx_modulation_type_t type)
Executes a continuous wave or modulation depending on the parameter type SIGFOX_API_stop_continuous_t...
sfx_u32 open_cs_frequency
Definition: sigfox_api.h:465
Definition: sigfox_api.h:442
Definition: sigfox_api.h:431
Definition: sigfox_api.h:353
Definition: sigfox_api.h:397
sfx_u32 open_rx_frequency
Definition: sigfox_api.h:481
Definition: sigfox_api.h:344
sfx_authentication_mode_t
Definition: sigfox_api.h:355
sfx_modulation_type_t
Definition: sigfox_api.h:399
Definition: sigfox_api.h:357
sfx_credentials_use_key_t
Definition: sigfox_api.h:448
Definition: sigfox_api.h:429
sfx_error_t SIGFOX_API_set_std_config(sfx_u32 config_words[3], sfx_bool timer_enable)
This function must be used to configure specific variables for standard. It is mandatory to call this...
Definition: sigfox_api.h:433
sfx_version_type_t
Definition: sigfox_api.h:346
sfx_error_t SIGFOX_API_switch_public_key(sfx_bool use_public_key)
Switch device on public or private key.
Definition: sigfox_api.h:419
Definition: sigfox_api.h:453
sfx_error_t SIGFOX_API_send_frame(sfx_u8 *customer_data, sfx_u8 customer_data_length, sfx_u8 *customer_response, sfx_u8 tx_mode, sfx_bool initiate_downlink_flag)
Send a standard SIGFOX frame with customer payload. Customer payload cannot exceed 12 Bytes.
Definition: sigfox_api.h:430
sfx_u32 macro_channel_width
Definition: sigfox_api.h:482
Definition: sigfox_api.h:395
sfx_rc_specific_t specific_rc
Definition: sigfox_api.h:485
sfx_delay_t
Definition: sigfox_api.h:437
sfx_error_t SIGFOX_API_stop_continuous_transmission(void)
Stop the current continuous transmission.
Definition: sigfox_api.h:399
Definition: sigfox_api.h:421
sfx_error_t SIGFOX_API_send_outofband(sfx_oob_enum_t oob_type)
Send an out of band SIGFOX frame which the type is passed as parameter of the function....
Definition: sigfox_api.h:393
Definition: sigfox_api.h:352
Definition: sigfox_api.h:394
Definition: sigfox_api.h:398
Definition: sigfox_api.h:420
sfx_spectrum_access_t spectrum_access
Definition: sigfox_api.h:484
sfx_error_t SIGFOX_API_receive_test_frame(sfx_u32 frequency, sfx_authentication_mode_t mode, sfx_u8 *buffer, sfx_u8 timeout, sfx_s16 *rssi)
This function waits for a valid downlink frame during timeout time and return in customer_data the da...
sfx_nvmem_t
Definition: sigfox_api.h:412
Definition: sigfox_api.h:404
sfx_spectrum_access_t
Definition: sigfox_api.h:336
sfx_modulation_type_t modulation
Definition: sigfox_api.h:483
Definition: sigfox_api.h:350
Definition: sigfox_api.h:358
Definition: sigfox_api.h:432
sfx_error_t SIGFOX_API_get_initial_pac(sfx_u8 *initial_pac)
Get the value of the PAC stored in the device. This value is used when the device is registered for t...
sfx_rf_mode_t
Definition: sigfox_api.h:425
sfx_u32 open_tx_frequency
Definition: sigfox_api.h:477
sfx_error_t SIGFOX_API_get_version(sfx_u8 **version, sfx_u8 *size, sfx_version_type_t type)
Returns current SIGFOX library version, or RF Version, or MCU version etc ..., in ASCII format ( depe...
Definition: sigfox_api.h:459
Definition: sigfox_api.h:441
sfx_s8 cs_threshold
Definition: sigfox_api.h:467
Definition: sigfox_api.h:444
Definition: sigfox_api.h:443
sfx_error_t SIGFOX_API_get_info(sfx_u8 *returned_info)
This function is to return info on send frame depending on the mode you're using. In DC and FH : re...
sfx_error_t SIGFOX_API_get_device_id(sfx_u8 *dev_id)
This function copies the ID of the device to the pointer given in parameter. The ID is ID_LENGTH byte...
sfx_error_t SIGFOX_API_open(sfx_rc_t *rc)
This function initialises library (mandatory). The SIGFOX_API_open function will :
Definition: sigfox_api.h:471
Definition: sigfox_api.h:342
Definition: sigfox_api.h:405
Definition: sigfox_api.h:452