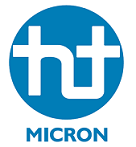 |
HT32SX Generic Push Button
Generic Push Button application for HT32SX
|
Go to the documentation of this file.
84 #define MONARCH_ERR_API_MALLOC (sfx_u8)(0x80)
85 #define MONARCH_ERR_API_FREE (sfx_u8)(0x81)
86 #define MONARCH_ERR_API_TIMER_START (sfx_u8)(0x82)
87 #define MONARCH_ERR_API_TIMER_STOP (sfx_u8)(0x83)
88 #define MONARCH_ERR_API_CONFIGURE_SEARCH_PATTERN (sfx_u8)(0x84)
89 #define MONARCH_ERR_API_STOP_SEARCH_PATTERN (sfx_u8)(0x85)
90 #define MONARCH_ERR_API_GET_VERSION (sfx_u8)(0x86)
178 sfx_error_t (* monarch_pattern_freq_result_callback_handler)(sfx_u32 freq,
sfx_pattern_enum_t pattern, sfx_s16 rssi));
sfx_u8 MONARCH_API_timer_stop(void)
This function stops the timer.
Definition: rf_api.c:2006
Definition: sigfox_monarch_api.h:90
sfx_u8 MONARCH_API_stop_search_pattern(void)
This function stops the scan.
Definition: rf_api.c:2013
sfx_pattern_enum_t
Definition: sigfox_monarch_api.h:72
sfx_u8 MONARCH_API_malloc(sfx_u16 size, sfx_u8 **returned_pointer)
Allocate memory for MONARCH library usage (Memory usage = size (Bytes)) This function is only called ...
Definition: rf_api.c:2000
sfx_u8 MONARCH_API_get_version(sfx_u8 **version, sfx_u8 *size)
This function returns current MONARCH API version.
Definition: rf_api.c:2015
sfx_monarch_listening_mode_t
Definition: sigfox_monarch_api.h:100
sfx_u8 MONARCH_API_free(sfx_u8 *ptr)
Free memory allocated to library with the MONARCH_API_malloc.
Definition: rf_api.c:2002
sfx_timer_unit_enum_t
Definition: sigfox_monarch_api.h:39
sfx_u8 MONARCH_API_configure_search_pattern(sfx_monarch_pattern_search_t list_freq_pattern[], sfx_u8 size, sfx_monarch_listening_mode_t mode, sfx_error_t(*monarch_pattern_freq_result_callback_handler)(sfx_u32 freq, sfx_pattern_enum_t pattern, sfx_s16 rssi))
This function is used to configure a search pattern action from on the MCU/RF side....
Definition: rf_api.c:2008
sfx_u8 MONARCH_API_timer_start(sfx_u16 timer_value, sfx_timer_unit_enum_t unit, sfx_error_t(*timeout_callback_handler)(void))
This function starts a timer based on timer_value and the units. When the timer expires the manufactu...
Definition: rf_api.c:2004