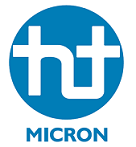 |
HT32SX Generic Push Button
Generic Push Button application for HT32SX
|
Go to the documentation of this file.
60 #define MCU_ERR_API_MALLOC (sfx_u8)(0x11)
61 #define MCU_ERR_API_FREE (sfx_u8)(0x12)
62 #define MCU_ERR_API_VOLT_TEMP (sfx_u8)(0x13)
63 #define MCU_ERR_API_DLY (sfx_u8)(0x14)
64 #define MCU_ERR_API_AES (sfx_u8)(0x15)
65 #define MCU_ERR_API_GETNVMEM (sfx_u8)(0x16)
66 #define MCU_ERR_API_SETNVMEM (sfx_u8)(0x17)
67 #define MCU_ERR_API_TIMER_START (sfx_u8)(0x18)
68 #define MCU_ERR_API_TIMER_START_CS (sfx_u8)(0x19)
69 #define MCU_ERR_API_TIMER_STOP_CS (sfx_u8)(0x1A)
70 #define MCU_ERR_API_TIMER_STOP (sfx_u8)(0x1B)
71 #define MCU_ERR_API_TIMER_END (sfx_u8)(0x1C)
72 #define MCU_ERR_API_TEST_REPORT (sfx_u8)(0x1D)
73 #define MCU_ERR_API_GET_VERSION (sfx_u8)(0x1E)
75 #define MCU_ERR_API_GET_ID_PAYLOAD_ENCR_FLAG (sfx_u8)(0x1F)
76 #define MCU_ERR_API_GET_PAC (sfx_u8)(0x20)
153 sfx_s16* temperature);
188 sfx_u8 *data_to_encrypt,
189 sfx_u8 aes_block_len,
sfx_u8 MCU_API_delay(sfx_delay_t delay_type)
Inter stream delay, called between each RF_API_send.
Definition: mcu_api_stm32.c:131
sfx_u8 MCU_API_get_nv_mem(sfx_u8 read_data[SFX_NVMEM_BLOCK_SIZE])
This function copies the data read from non volatile memory into the buffer pointed by read_data....
Definition: mcu_api_stm32.c:74
sfx_u8 MCU_API_set_nv_mem(sfx_u8 data_to_write[SFX_NVMEM_BLOCK_SIZE])
This function writes data pointed by data_to_write to non volatile memory. It is strongly recommande...
Definition: mcu_api_stm32.c:85
sfx_u8 MCU_API_timer_start(sfx_u32 time_duration_in_s)
Start timer for in second duration.
Definition: tim.c:284
sfx_u8 MCU_API_timer_start_carrier_sense(sfx_u16 time_duration_in_ms)
Start timer for :
Definition: tim.c:228
sfx_u8 MCU_API_malloc(sfx_u16 size, sfx_u8 **returned_pointer)
Allocate memory for library usage (Memory usage = size (Bytes)) This function is only called once at ...
Definition: mcu_api_stm32.c:53
sfx_u8 MCU_API_get_voltage_temperature(sfx_u16 *voltage_idle, sfx_u16 *voltage_tx, sfx_s16 *temperature)
Get voltage and temperature for Out of band frames Value must respect the units bellow for backend co...
Definition: mcu_api_stm32.c:104
sfx_credentials_use_key_t
Definition: sigfox_api.h:448
sfx_u8 MCU_API_aes_128_cbc_encrypt(sfx_u8 *encrypted_data, sfx_u8 *data_to_encrypt, sfx_u8 aes_block_len, sfx_u8 key[16], sfx_credentials_use_key_t use_key)
Encrypt a complete buffer with Secret or Test key. The secret key corresponds to the private key pro...
Definition: mcu_api_stm32.c:155
sfx_u8 MCU_API_get_device_id_and_payload_encryption_flag(sfx_u8 dev_id[ID_LENGTH], sfx_bool *payload_encryption_enabled)
This function copies the device ID in dev_id, and the payload encryption flag in payload_encryption_e...
Definition: mcu_api_stm32.c:36
sfx_u8 MCU_API_report_test_result(sfx_bool status, sfx_s16 rssi)
To report the result of Rx test for each valid message received/validated by library....
Definition: mcu_api_stm32.c:180
sfx_delay_t
Definition: sigfox_api.h:437
sfx_u8 MCU_API_get_version(sfx_u8 **version, sfx_u8 *size)
Returns current MCU API version.
Definition: mcu_api_stm32.c:172
sfx_u8 MCU_API_free(sfx_u8 *ptr)
Free memory allocated to library.
Definition: mcu_api_stm32.c:96
sfx_u8 MCU_API_timer_stop_carrier_sense(void)
Stop the timer (started with MCU_API_timer_start_carrier_sense)
Definition: tim.c:345
sfx_u8 MCU_API_timer_stop(void)
Stop the timer (started with MCU_API_timer_start)
Definition: tim.c:333
sfx_u8 MCU_API_get_initial_pac(sfx_u8 initial_pac[PAC_LENGTH])
Get the value of the initial PAC stored in the device. This value is used when the device is register...
Definition: mcu_api_stm32.c:45
sfx_u8 MCU_API_timer_wait_for_end(void)
Blocking function to wait for interrupt indicating timer elapsed. This function is only used for the...
Definition: tim.c:371